Getting Started with the Tayza Deliver API
Getting your API Key
To use the Tayza Deliver API, you first need to get your hands on an API key. You can find your API key on your Tayza client portal, under settings. without an API key, the majority of the API's functions are inaccessible.
Tayza's delivery service is currently closed-registration only. If you aren't a Tayza user (but would like to be), get in contact with sales and we will be able to create an account for you.
Signing your Requests
In order to use your API key to authenticate requests, you'll need to set an additional
request header for requests sent to the API endpoint. if this is not done, all requests will return 401: Unauthorized
codes
in their response. the following header needs to be added:
X-API-Key: <Your API Key>
HTTPS/SSL
When using the Deliver API in production, your are required to send requests over HTTPS, and failure to do so will be considered a breach of our terms of service.Checking your API Key
Before proceeding into using the Deliver API, it is important to check that your API key is valid and associated
with your Tayza Account. to check if your API key is valid, use the following endpoint, with the X-API-Key
header attached to your request.
A valid key will produce a 200: OK
status code return further auth data, including account ID and Company Name
If the key is invalid, you will receive either a 401: Unauthorized
or 403: Forbidden
response.
Example: Valid Key
GET https://deliver.tayza.io/me
status: 200 OK
body: {
message: 'Valid user',
authData: {
userID: <Your User ID>,
companyName: <Your Company Name>,
apiKey: <Your API Key>
}
}
Creating a Delivery
To create a new delivery, you need to define objects for recipient
, address
,
and options
. The endpoint will respond with a 201 Created
response if a
delivery is successful, and 400 Bad Request
response if the information entered is invalid.
Common sources of failed creation include non-geocodeable addresses and invalid phone numbers.
When sending the POST
request, send a composed delivery
object consisting of the three sub-objects mentioned above. For the address
object, the fields for unit, number, and street
can be replaced with a single address.line1
field. All fields for delivery creation accept strings, except for pickupIndex
and giftSender
.
Example
POST https://deliver.tayza.io/deliveries
body: {
delivery: {
recipient: {
name: <recipient name>,
phone: <phone number?>
},
address: {
unit: <unit?>,
number: <street number>,
street: <street name>,
city: <city>,
province: <ON>,
country: <Canada>,
postalCode: <postal code>
},
options: {
quantity: <number of packages>,
deliveryNotes: <notes?>,
giftSender: <gift sender?>,
pickupIndex: <pickup address # on account || null for default address>
}
}
}
status: 201 Created
body: {
message: 'Delivery successfully created'
data: {
_id:<Delivery ID>,
client: {
_id:<Client ID>,
companyName:<Client company name>,
address: {
unit: <unit?>,
number: <street number>,
street: <street name>,
city: <city>,
province: <ON>,
country: <Canada>,
postalCode: <postal code>
}
},
recipient: {
name: <recipient name>,
phone: <phone number?>
},
address: {
unit: <unit?>,
number: <street number>,
street: <street name>,
city: <city>,
province: <ON>,
country: <Canada>,
postalCode: <postal code>
},
options: {
quantity: <number of packages>,
deliveryNotes: <notes?>,
giftSender: <gift sender?>,
pickupIndex: <pickup address # on account || null for default address>
},
status: {
submitted: <ISO Date>,
active: <ISO Date or undefined>,
inProgress: <ISO Date or undefined>,
completed: <ISO Date or undefined>,
failed: <ISO Date or undefined>
}
}
}
Getting a Delivery by ID
To get a specific delivery by its ID, send a GET
request to the deliveries
endpoint, with the delivery ID appended to the end. The response body will contain the fetched delivery if found
or will return a 404 Not Found
response if no such delivery exists.
Example
GET https://deliver.tayza.io/deliveries/<id>
status: 200 OK
body: {
message: 'Delivery found'
data: {
_id:<Delivery ID>,
client: {
_id:<Client ID>,
companyName:<Client company name>,
address: {
unit: <unit?>,
number: <street number>,
street: <street name>,
city: <city>,
province: <ON>,
country: <Canada>,
postalCode: <postal code>
}
},
recipient: {
name: <recipient name>,
phone: <phone number?>
},
address: {
unit: <unit?>,
number: <street number>,
street: <street name>,
city: <city>,
province: <ON>,
country: <Canada>,
postalCode: <postal code>
},
options: {
quantity: <number of packages>,
deliveryNotes: <notes?>,
giftSender: <gift sender?>,
pickupIndex: <pickup address # on account || null for default address>
},
status: {
submitted: <ISO Date>,
active: <ISO Date or undefined>,
inProgress: <ISO Date or undefined>,
completed: <ISO Date or undefined>,
failed: <ISO Date or undefined>
}
}
}
Getting all Current Deliveries
Fetches all current deliveries - defined as any delivery that is currently incomplete or has been completed within the current day. When operating at a high capacity, it may be more performant to use the list fetch in place of getting all current deliveries at once.
GET https://deliver.tayza.io/deliveries/current
status: 200 OK
body: {
message: 'Current deliveries successfully fetched'
data: [
{DeliveryObject_1},
{DeliveryObject_2},
...
{DeliveryObject_n}
]
}
Getting a Paginated List of Deliveries
Fetches a list of delivery objects of length limit
if defined, otherwise will fetch
a default length of 20 items. The deliveries are sorted in reverse chronological order (newest first).
The page
parameter allows for skipping ahead (page*limit)
entries, and allows
for pagination for the deliveries list. The page is zero-indexed, with page=0
containing
the most recent deliveries.
The response also includes a numPages
field, which indicates the total number of pages
available at the provided limit
size. much like in arrays, the final page will be equal to
numPages - 1
.
GET https://deliver.tayza.io/deliveries/list/<page>/<limit?>
status: 200 OK
body: {
message: 'Current deliveries successfully fetched'
data: {
deliveries: [
{DeliveryObject_1},
{DeliveryObject_2},
...
{DeliveryObject_limit || DeliveryObject_20}
]
numPages: <total pages in list>
}
}
Deleting a Delivery by ID
To delete a specific delivery by its ID, send a DELETE
request to the deliveries
endpoint, with the delivery ID appended to the end. The response body will contain the deleted delivery if found
or will return a 404 Not Found
response if no such delivery exists.
Deliveries cannot be deleted if they have been dispatched (if its most recent status is inProgress
,
completed
, or failed
), and attempting to delete a delivery of this class will
yield a 400 Bad Request
response.
DELETE https://deliver.tayza.io/deliveries/<id>
status: 200 OK
body: {
message: 'Delivery successfully removed',
data: {
_id:<Delivery ID>,
client: {
_id:<Client ID>,
companyName:<Client company name>,
address: {
unit: <unit?>,
number: <street number>,
street: <street name>,
city: <city>,
province: <ON>,
country: <Canada>,
postalCode: <postal code>
}
},
recipient: {
name: <recipient name>,
phone: <phone number?>
},
address: {
unit: <unit?>,
number: <street number>,
street: <street name>,
city: <city>,
province: <ON>,
country: <Canada>,
postalCode: <postal code>
},
options: {
quantity: <number of packages>,
deliveryNotes: <notes?>,
giftSender: <gift sender?>,
pickupIndex: <pickup address # on account || null for default address>
},
status: {
submitted: <ISO Date>,
active: <ISO Date or undefined>,
inProgress: <ISO Date or undefined>,
completed: <ISO Date or undefined>,
failed: <ISO Date or undefined>
}
}
}
Generating Delivery Labels (PDF)
To get labels for a list of deliveries, you need to submit a comma-separated string of delivery IDs
for the IDs
query parameter of your request. You can optionally include a parameter
for thermal
, which, if true, will generate labels optimized for 4x6 thermal label printers.
Otherwise, the labels will be generated four-a-page for 8.5x11 letter paper.
The endpoint responds with a Blob
with content type equal to
application/pdf
. You will need to work with this Blob
locally in order to
convert it to a usable PDF file.
See also: Converting Blob data to files using Javascript and createObjectURL()
GET https://deliver.tayza.io/deliveries/labels?ids=<id_1,id_2,id_3,...,id_n>[&thermal=<bool?>]
status: 200 OK
body: {Blob of content-type: application/pdf}
Generating Delivery Labels (ZPL)
To get labels for a list of deliveries, you need to submit a comma-separated string of delivery IDs
for the IDs
query parameter of your request. The labels generated are optimized for
4x6 thermal paper printers. A parameter for dpmm
can be provided for the dpmm of your printer: 6, 8, 12 and 24 dpmm
printers are supported. 8 dpmm is the default for the labels returned if no parameter is provided.
The endpoint responds with an array with each element containing an array of strings. Each element in the data array
contains one or more strings corresponding to an individual label per package as defined by delivery.quantity
.
GET https://deliver.tayza.io/deliveries/labels/zpl?ids=<id_1,id_2,id_3,...,id_n>[&dpmm=<6||8||12||24?>]
status: 200 OK
body: {
data: [
[Delivery_1Pkg1, Delivery1Pkg2, ...]
[Delivery_2Pkg1, ...]
...
[Delivery_NPkg1, ...]
]
}
Getting a List of Delivery Zones
Returns a list of zone
objects, which contain the zone ID, title, price to fulfill, and a list of FSAs
(the first three characters in a Canadian Postal Code) serviced by that zone. This list can be used to estimate price
by location and paints a picture of the total area serviced by Tayza.
GET https://deliver.tayza.io/zones
status: 200 OK
body: {
message: 'Zone list fetched',
data: [
{
_id:<Zone ID>,
title:<Name of zone_1>,
price:<Cost to deliver to zone>,
postalCodes: [<FSA_1_1>,<FSA_1_2>,<FSA_1_3>,...,<FSA_1_n>]
},
{
_id:<Zone ID>,
title:<Name of zone_2>,
price:<Cost to deliver to zone>,
postalCodes: [<FSA_2_1>,<FSA_2_2>,<FSA_2_3>,...,<FSA_2_n>]
},
{
_id:<Zone ID>,
title:<Name of zone_3>,
price:<Cost to deliver to zone>,
postalCodes: [<FSA_3_1>,<FSA_3_2>,<FSA_3_3>,...,<FSA_3_n>]
},
...
{
_id:<Zone ID>,
title:<Name of zone_n>,
price:<Cost to deliver to zone>,
postalCodes: [<FSA_m_1>,<FSA_m_2>,<FSA_m_3>,...,<FSA_m_n>]
}
]
}
Getting Estimated Shipping Rates for a Package
Returns a data
object which contains a message and a data object.
The inner data
object contains the zone the delivery falls under,
the package type applied to the delivery, the delivery rate, and the postal code provided.
A valid postal code will produce a 200: OK
status code and return
relevant shipping data. If the postal code is invalid or out of range for the delivery service
you will receive either a 400: Bad request
or 404: Not found
response respectively with the error message
included in the response. This is also true
If the package dimensions are invalid/incomplete or outside of any package category, and will
include error messages reflecting as such.
A valid postal code will include:
- Characters formated A1A1A1 after formatting
- exactly 6 characters for exact matching, or 4-6 characters ending with the *
symbol for partial matching
Example: Valid Postal Code
POST https://deliver.tayza.io/zones/rate
body: {
postalCode: <canadian postal code [Format A1A1A1]>
dimensions: {
length: <Length [Metres]>
width: <Width [Metres]>
height: <Height [Metres]>
weight: <Weight [Kilograms]?>
}
}
status: 200 OK
body: {
message: 'Valid delivery',
data: {
zone: <Name of zone>,
packageType: <Package type name>,
postalCode: <Postal code>,
price: <Delivery rate>
}
}
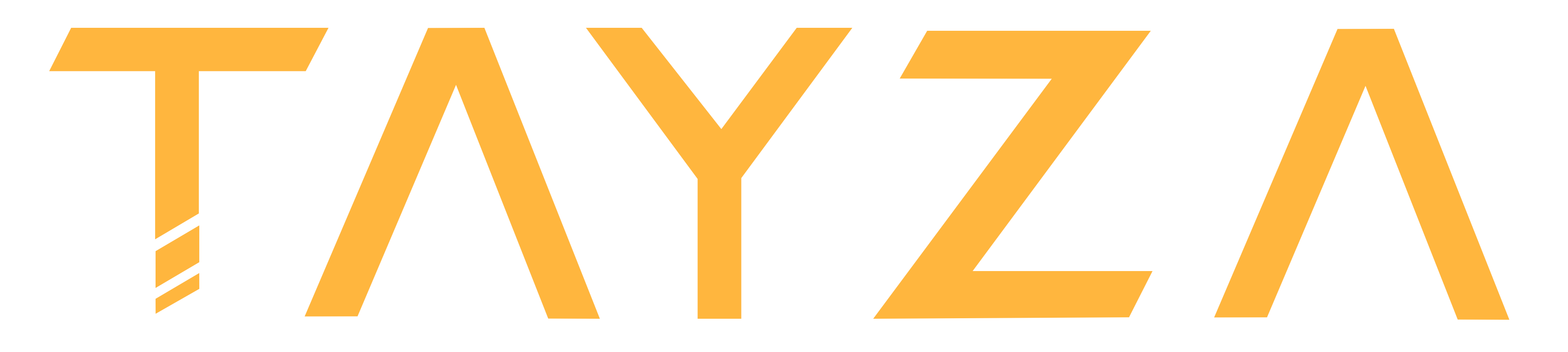